Mastering the System Designs Interview for Apps Developers
Welcome to the Interview Loop series where we go over each step of your interview loop.
- Intro to the Apps Developer Interview Loop
- Initial Phone Screen
- Technical Phone Screen
- The Coding Exercise
- The Algorithm Interview
- The System Designs Interview
- The App Specialty Interview
- The Hiring Manager Closing Interview
Remember, while some job interviews may include all these sessions, others might involve a subset. Prepare accordingly.
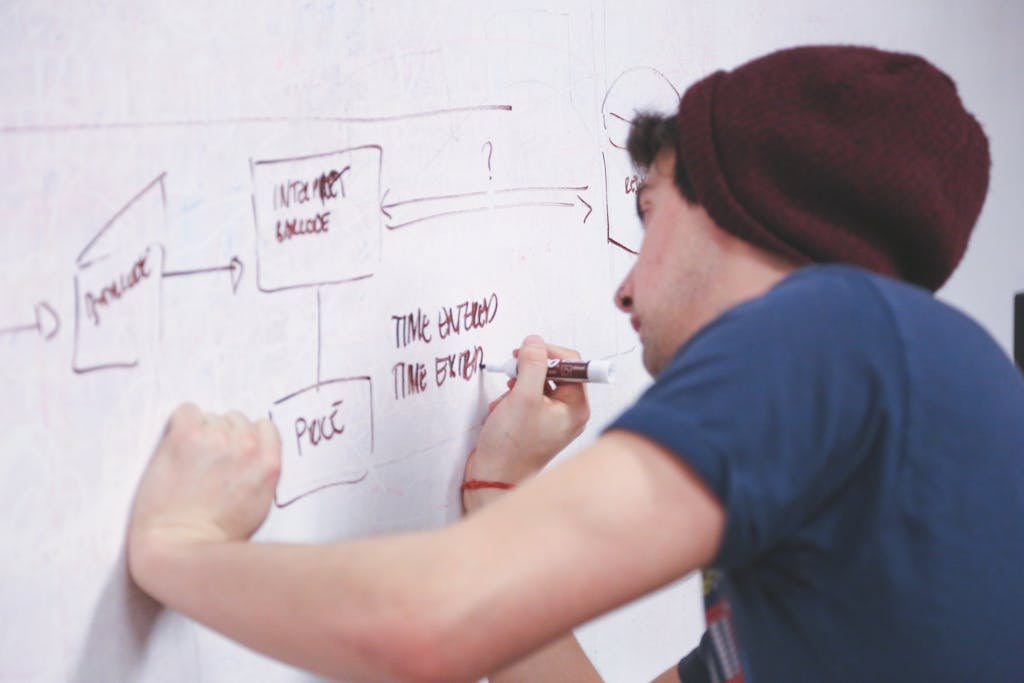
The System Design interview for Mobile Apps Developers is a pretty common one.
Whether you’re building Android, iOS, Flutter or React Native apps, there’s a good chance you’ve already encounter this type of questions before.
And while I find the algorithm interview pretty irrelevant for apps developers, the system designs interview reveals a lot about you. From your communication skills to experience and problem solving, you better be prepared for what’s to come.
The system designs interview isn’t the most difficult of the Apps Developer Interview Loop, yet many get it wrong by throwing buzz words they don’t understand (like CLEAN architecture) or jumping into the solution without asking enough questions.
In this guide, we’ll go over a few common problems, what questions you need to ask to properly define the scope of the exercise, and bullet proof scalable solutions to answer like an expert.
Common Problems
System Design interviews are such a good tool for employers to gauge the level of a developer that you can expect it from big FAANG type companies (Facebook, Amazon, Apple, Netflix and Google) to the smallest startups.
The System Design interview for Apps Developers typically focuses on how you would design and architect a mobile app. While bigger companies sometimes go beyond and want to see how you would think about broader systems and touch on backend micro-services (even if you’ve never worked with them), we’ll focus on a few mobile specific problems you can encounter.
- Design a communication product (e.g. WhatsApp, Slack)
- Design a social network (e.g. Facebook, Instagram)
- Design the interviewing company’s mobile app (Productivity, Finance, Ridesharing etc…)
The secret is that while these apps are pretty different in functionalities, there is a lot of overlap in the technical challenges required to make them scalable and offline first. Therefore, you can follow the following framework to get the interview right 100% of the time.
- Prepare: As always, preparation will get you a long way. Start by researching the company’s typical System Design questions on Glassdoor to see if you can find what to expect. Also be prepared to answer any of the common encounters listed above. Finally, take the time to think about how you would design the company’s app. Never go into the interview blind.
- Define the scope and ask questions: The most common mistake people do during this interview is to jump right into solutions before understanding the scope of the exercise. Listen to the interviewer carefully, understand what they expect from you, and ask the right questions (more on this below)
- Lay out a high level architecture: Once the scope is clear, lay out the right architecture. This is a lot more about your data layer than how you build your UI. Most companies will expect you to describe a scalable architecture to millions of users and dozens of developers, though some early stage startups will be more interested in the fastest path to market. Always make sure that you describe an architecture specific to the scope of the question.
- Follow the interviewer’s lead to go deeper: There is no need to ramble and talk for 20 minutes about your perfect architecture. Start by touching on all the points your interviewer is interested about, then let him/her take the lead on where to bring it next. Often times, this means going deeper into your data layer, data integrity and stream architecture. Don’t spend 10 minutes talking about how you build a UI widget, this is not what this is about.
Define the scope
Start by making sure you understand exactly what’s expected from you. Never start answering a System Designs problem without asking the right questions. Your interviewer isn’t only interested in the final answer. He wants to know how you communicate, scope the exercise and problem solve. Before jumping in, always make sure the scope of the exercise is fully defined.
Here are some basic questions you should always ask in order to narrow it down to what your interviewer wants to hear.
- Who are the target users of the app and how many users should I expect?
Understanding the audience is a good way to define the tradeoffs you will want to make when describing your architecture. If you’re asked to build an app like Messenger that will be downloaded by billions of users, you’ll need to take an offline first, scalable approach. It could also be that your interviewer is more interested in hearing how you’d build a chat support feature for an early stage product, in which case you should prioritize a speedy implementation over a complex architecture. - What are the functional requirements?
There is a lot of nuance to different apps, and always be careful to understand what’s expected from you. Contrary to other messaging products, WhatsApp doesn’t rely on an API as much and stores messaging on device, and has a sync protocol to synchronize across different devices. Get into the nitty gritty of the product and make sure you touch on everything that is unique to the app you’re asked to design. Be sure to understand the type of API you need to integrate with what type of offline support you’re expected to describe. - What are the non-functional requirements?
Beyond functionalities, you should be thinking of how the app is supposed to perform, the security requirements (api key/data encryption, secure communications) and the supported platforms (iOS, Android, cross-platform). Interviewers don’t always go deeper into these subjects but they expect you to bring them up early on. Be ready to describe how you would encrypt an API key and what cross-platform solutions could look like beyond your expertise. - What is the budget and timeline?
The job of an apps developer is not only to build stuff, but also to balance tradeoffs. If you’re talking to a 3 people startups, they probably don’t want to hear how you would build a 1 billion user product. Make sure you discuss how many developers would be expected on the project (hypothetically) and how quickly it needs to come together so you can discuss what shortcuts could be taken to bring the product to market quicker.
You should be spending anything between 2 and 5 minutes asking questions before answering anything. Once the scope is fully defined, we can start talking architecture.
Scalable architecture
With a clear scope in mind, you should now be ready to propose an architecture that matches your interviewer’s expectations. Remember, this interview is not about how you would build UI widgets- interviewers often care about more complex problems such as offline support, data integrity and a clean separation of concerns.
Don’t just throw buzz words out there. Talking about CLEAN Architecture right away is usually a red flag because it’s vague and means different things to different people. Instead, be specific about the main building blocks of your application and how they interact with each other.
Let’s dive into what mobile apps system designs often look like in 2024 for complex apps.
Mobile Apps System Architecture
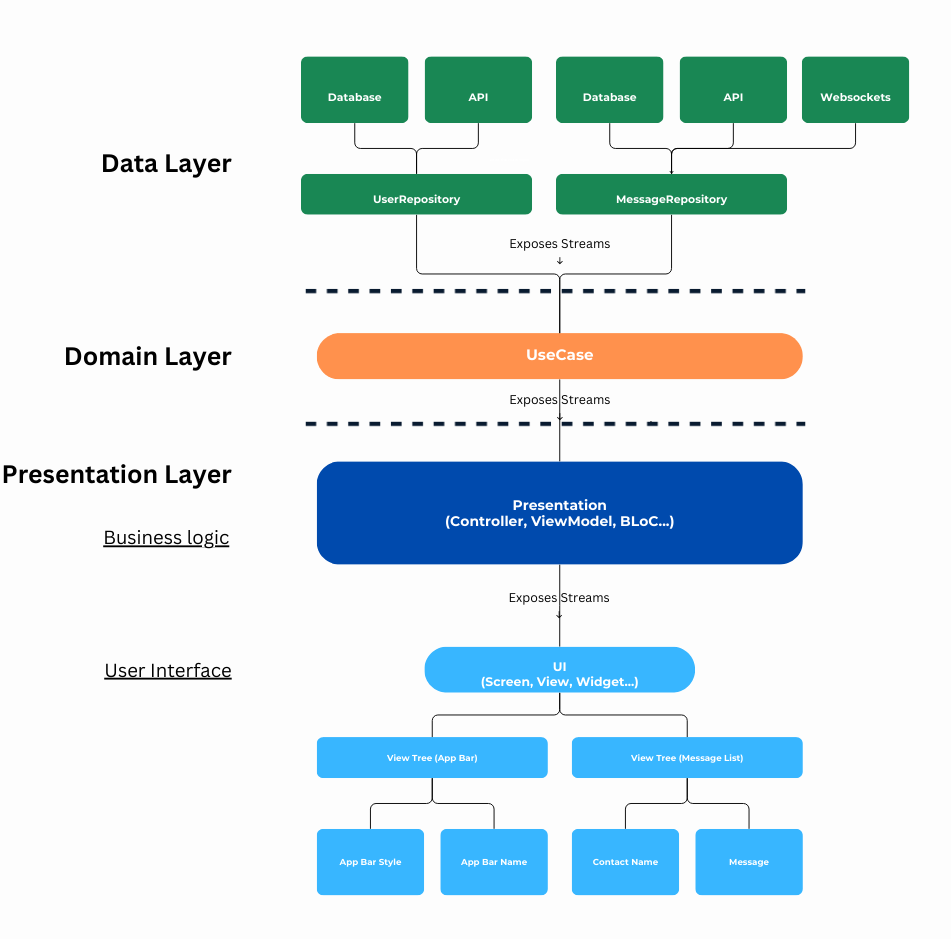
Reactive Design
Modern apps are highly reactive, and often leverage Streams and a robust Data Layer in order to achieve high performance and data integrity. The way this works is pretty simple: your app’s data is stored in your data layer, and subsequent layers listen to and transform streams of data all the way down to your UI.
For this to work, you typically need to leverage one of the many stream libraries available in different mobile apps environment. Just to name a few:
iOS: Combine, RxSwift
Android: Coroutines, RxJava
Flutter: RxDart
React Native: RxJs
Data Layer
In the diagram above, you can see that the Data Layer is exposed to your application through Repositories which are fueled by different Data Sources (Local/Database, Remote/API/Websocket…). The benefit of this abstraction is that it allows to easily swap out a database or an API, and maintaining different repositories for different areas of an API makes migrations a lot simpler. Typically, the role of your repository is to be the glue between these data sources and exposes the final data to your domain or presentation layer.
Your Data Layer is often the crux of the System Designs interview. Not only it’s the easiest layer to get wrong, but it’s also the most consequential as its behavior trickles down to the rest of your application.
Here, the separation of concerns is pretty straightforward and most developers nowadays are able to describe it accurately. Just keep in mind that while multiple repositories are great for complex applications, they can be overkill for simpler ones- make sure you take the exercise’s scope into account.
But there is one thing this type of diagram doesn’t cover: your data integrity.
One of the biggest challenges of building scalable mobile apps is to properly balance your online and offline data in a way that is simple, efficient and reliable. Let’s zoom into a common approach to solving this problem.
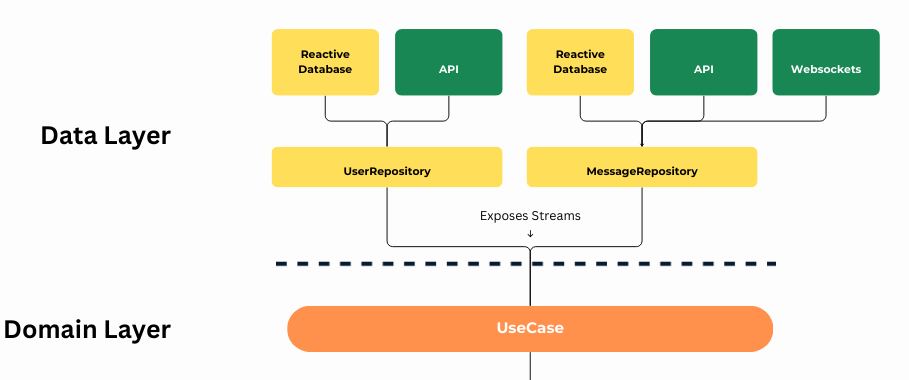
The common pitfall for apps developers is to manage their online/offline data mediation manually in the repository, and swapping data sources based on the network state.
While this works in theory, it introduces a lot of complexity and performance challenges.
There is however a much simpler way to solve this problem: using a reactive database as your source of truth.
A reactive database is like a regular database except that you can turn your queries into observable streams. This allows you to get an updated list of your content as various CRUD (Create, Read, Update, Delete) operations happen both on device and on the API side.
The simplest solution to this problem is to update your local database for both local and remote operations, and listen to databases updates to show your content rather than network status and responses. In practice, this means that your Repositories should mostly just stream database updates for a given queries (for example, query all the messages for a given conversation id) and only expose network data for network specific operations (for example login success/failure).
Master the data layer and you’ll have a good shot at nailing this interview.
Domain Layer (Optional)
The domain layer consists of UseCases classes and is an optional architecture layer that can be very useful when dealing with different repositories.
A UseCase is a single responsibility class that sits between your Data Layer and your presentation layer. If fulfills a single functionality or interaction in the system from a user’s perspective and has three main benefits that you can bring up to your interviewer.
- Business logic reusability
If you find yourself reusing the exact business same logic in multiple Controllers/ViewModels/BLoCs etc…, you could extract this logic into a simple UseCase class instead. - Single purpose repositories
UseCase classes allow to keep repositories decoupled from each other by moving the coupling logic into a UseCase rather than the repository itself. This typically allows application to scale and handle refactors better. - Testability
A single piece of logic is typically a lot easier to test than when it is embedded in your presentation layer.
Once again, remember to take the exercise’s scope into account. While multi-repository applications can greatly benefit from UseCases, it’s completely overkill for single repo ones. Apps developers often get extra points for understanding the domain layer in complex applications.
Presentation Layer
Finally, the Presentation Layer is responsible for your Business Logic and User Interface. Modern app development frameworks are often enforcing this separation of concerns so you don’t mix business logic and UI (SwiftUI, Jetpack Compose, Flutter and React are all pretty good at this).
Typically, interviewers won’t linger on this layer because it is the most straightforward. This is also probably the layer you’re the most familiar with anyway. Just in case, here’s a quick reminder.
- Business Logic
Your business logic should be entirely encapsulated in your controller (react aside) and create states to emit to your view - User Interface / View
Your view should know nothing about your business logic and simply react to views emitted by your controller
While you may not get many questions on this, know the separation of concerns well, and be ready to talk about specific view behaviors such as how you would populate a list.
Conclusion
This should give you a pretty good understanding of what is expected of you during System Designs interviews for app developers.
Remember, never rush into an answer and always scope things first. Be communicative with your interviewer and ensure this is a two-way conversation. Then don’t wave over the details- talk architecture and data because that’s what other engineers want to hear from you.
If some of these concepts aren’t familiar to you, I would suggest you go build a simple project to play around with them and understand them deeply. These have now become industry standards and you’re expected to understand the basic principles of a reactive app architecture.